How to color table using CSS
The previous chapter covered how to change the basic styles of the table using CSS. In this chapter we are going to a give more styles to the tables using CSS. Once you create the structure of the table in the markup, its easy to adding a layer of style to customize its appearance.
CSS Table Background color
The CSS background-color property allows you to color background of a table, row and cells.
tr
{
background-color:green;
color:white;
}
The above code color the background of each row as green color and foreground color as white.
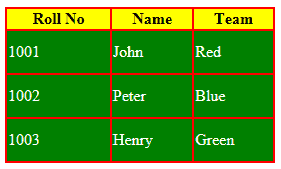
Source Code
<!DOCTYPE html>
<html>
<head>
<style>
table,th,td
{
border:2px solid red;
}
table
{
border-collapse:collapse;
width:20%;
}
td
{
height:40px;
}
tr
{
background-color:green;
color:white;
}
th
{
background-color:yellow;
color:black;
}
</style>
</head>
<body>
<table>
<tr>
<th>Roll No</th>
<th>Name</th>
<th>Team</th>
</tr>
<tr>
<td>1001</td>
<td>John</td>
<td>Red</td>
</tr>
<tr>
<td>1002</td>
<td>Peter</td>
<td>Blue</td>
</tr>
<tr>
<td>1003</td>
<td>Henry</td>
<td>Green</td>
</tr>
</table>
</body>
</html>
How to color specific row in a CSS Table
You can use the tr:nth-child(rownumber) to color a particular row in a table using CSS.
tr:nth-child(3)
{
background-color: green;
color:white;
}
Above code select the 3 row from top (including table head row) and color background as green and foreground as white.
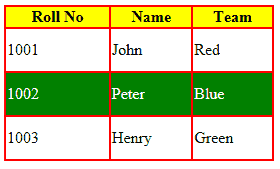
CSS Code
<style>
table,th,td
{
border:2px solid red;
}
table
{
border-collapse:collapse;
width:20%;
}
td
{
height:40px;
}
tr:nth-child(3)
{
background-color: green;
color:white;
}
th
{
background-color:yellow;
color:black;
}
</style>
Applied this CSS code to the Example 1 HTML Table
How to color specific column in a CSS Table
You can give background color to specific column by suing td:nth-child(columnnumber) .
td:nth-child(1)
{
background-color: orange;
}
Above code color the first coloumn background color as Orange.
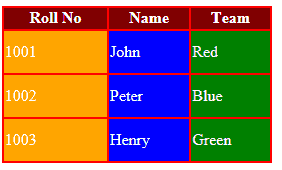
CSS Code
<style>
table,th,td
{
border:2px solid red;
color:white;
}
table
{
border-collapse:collapse;
width:20%;
}
th
{
background-color: maroon;
}
td
{
height:40px;
}
td:nth-child(1)
{
background-color: orange;
}
td:nth-child(2)
{
background-color: blue;
}
td:nth-child(3)
{
background-color: green;
}
</style>
Applied this CSS code to the Example 1 HTML Table
How to color CSS Table cell only
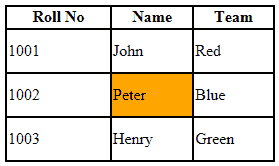
The following source code shows how to color a particular cell in a table using CSS.
<!DOCTYPE html>
<html>
<head>
<style>
table,th,td
{
border:2px solid black;
}
table
{
border-collapse:collapse;
width:20%;
color:black;
}
td
{
height:40px;
}
#orange
{
background-color: orange;
}
</style>
</head>
<body>
<table>
<tr>
<th>Roll No</th>
<th>Name</th>
<th>Team</th>
</tr>
<tr>
<td>1001</td>
<td>John</td>
<td>Red</td>
</tr>
<tr>
<td>1002</td>
<td id="orange">Peter</td>
<td>Blue</td>
</tr>
<tr>
<td>1003</td>
<td>Henry</td>
<td>Green</td>
</tr>
</table>
</body>
</html>
CSS Table Alternate row coloring
You can use tr:nth-child(rowOrder) to give alternating row color to a Table using CSS. The rowOrder must be “odd” or “even”.
tbody tr:nth-child(even)
{
background: orange;
}
Above code color every even row order to background color as orange.
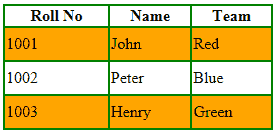
CSS Code
<style>
table,th,td
{
border:2px solid green;
}
table
{
border-collapse:collapse;
width:20%;
}
td
{
height:30px;
}
tbody tr:nth-child(even)
{
background: orange;
}
</style>
Applied this CSS code to the Example 1 HTML Table
For CSS table alternate column coloring you can use the CSS code like the following.
tbody td:nth-child(even)
{
background: orange;
}
Above code color alternate column to Orange background.
CSS Table Color first column and first row
Using a simple technique, you can color the first row and first column of a CSS table.
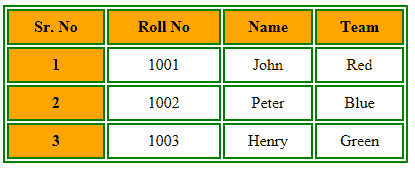
Full Source
<!DOCTYPE html>
<html>
<head>
<style>
th
{
background: orange;
height:30px;
}
table,th,td
{
border:2px solid green;
}
table
{
width:30%;
color:black;
text-align:center;
}
</style>
</head>
<body>
<table>
<tr>
<th>Sr. No</th>
<th>Roll No</th>
<th>Name</th>
<th>Team</th>
</tr>
<tr>
<th>1</th>
<td>1001</td>
<td>John</td>
<td>Red</td>
</tr>
<tr>
<th>2</th>
<td>1002</td>
<td>Peter</td>
<td>Blue</td>
</tr>
<tr>
<th>3</th>
<td>1003</td>
<td>Henry</td>
<td>Green</td>
</tr>
</table>
</body>
</html>